C++ If-else statements in c++ are the conditional statements. They are used to decide which part or code block of the program will be executed according to the pre-defined conditions true or false. Relational operators are used to specify the conditions in c++.
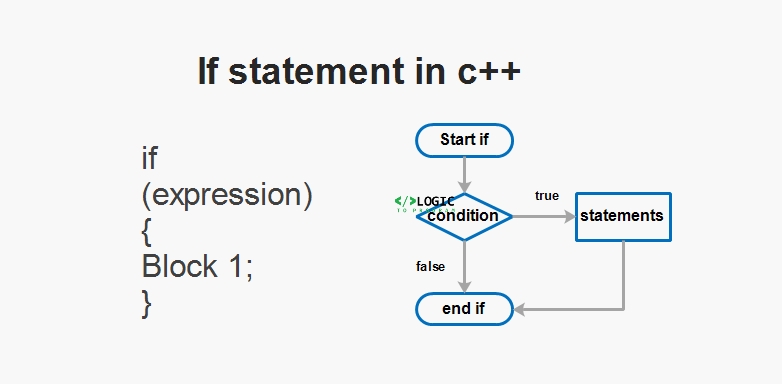
There are many ways to use if-else statements in C++ like if, if-else, if-else-if, and nested if. Here we discuss them one by one.
If Statement in C++
Only if statement in C++ is used to execute or skip a block of statements based on the condition is true or false.
Syntax: if statement in C++
If (expression)
{
statement;
}
Flow chart: if statement in C++
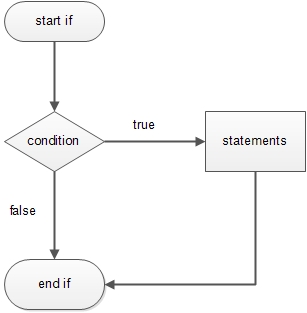
Example: if statement in C++
//simple if Statement in C++
#include <iostream>
using namespace std;
int main()
{
int number = -1;
// If number is greater then 0 execute if block
if (number > 0) {
cout << "Your are right"<< endl;
}
cout << "You are out of if block";
return 0;
}
Output
if the number is equal to 4, it means the value is greater than 0 so the output will be
Your are right
You are out of if block
if the number is equal to -1, it means the value is less than 0 so that if condition is terminated and control goes outside the if statement. The output will be.
You are out of if block
If-else Statement in C++
If-else statement in C++ is used to execute one block of the statement when the condition is true and the other block of statements when the condition is false. In an if-else statement, both blocks of statements can never be executed and skipped at once.
Syntax: if-else statement in C++
If (expression)
{
statements;
}
Else
{
statements;
}
Flow chart: if statement in C++

Example: if-else statement in C++
//simple if Statement in C++
#include <iostream>
using namespace std;
int main()
{
int number = -1;
/* If number is greater then 0 execute if block
otherwise execute else part */
if (number > 0) {
cout << "Your are in the if part"<< endl;
}
else
{
cout << "You are in the else part";
}
return 0;
}
Output
If the number is greater than 0 execute if block otherwise execute else part
You are in the else part
If-else-if statement in C++
If-else-if statement in C++ is used to execute one block of statements from many blocks based on the true condition.
Syntax: if-else-if statement in C++
If(condition)
{
statements 1;
}
else if(condition)
{
statements 2;
}
else if(condition)
{
statements 3;
}
Else
{
statements N;
}
Flow chart: flow chart of if-else-if statement in C++
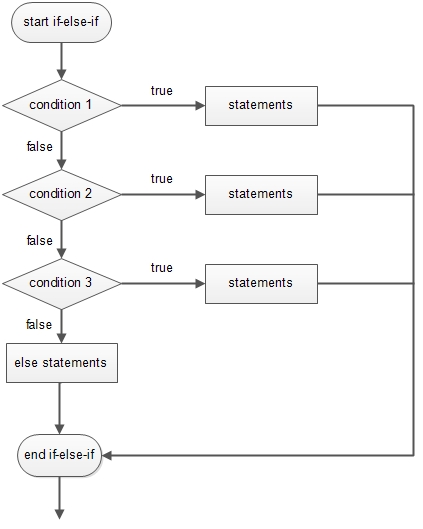
Example: if-else-if statement in c++
//if-else-if Statement in C++
#include <iostream>
using namespace std;
int main()
{
int number1, number2;
cout<<"Input the value of var1:"<<endl;
cin>>number1;
cout<<"Input the value of var2:"<<endl;
cin>>number2;
if (number1 > number2)
{
cout<<"number1 is greater than number2"<<endl;
}
else if (number2 > number1)
{
cout<<"number2 is greater than number1"<<endl;
}
else
{
cout<<"number1 is equal to number2"<<endl;
}
return 0;
}
Output 1
Input the value of var1:
8
Input the value of var2:
3
number1 is greater than number2
Output 2
Input the value of var1:
3
Input the value of var2:
8
number2 is greater than number1
Output 3
Input the value of var1:
6
Input the value of var2:
6
number1 is equal to number2
Nested if statement in C++
In the Nested if statement, the control enters the inner part only when the outer if condition is true. Users can use multiple if-else-if statements inside the if statement as required.
Syntax: Nested if statement in C++
If (condition)
{
If(condition)
{
Statements
}
Else
{
Statements
}
}
Else
{
Statements
}
Flow chart: Nested if statement in c++
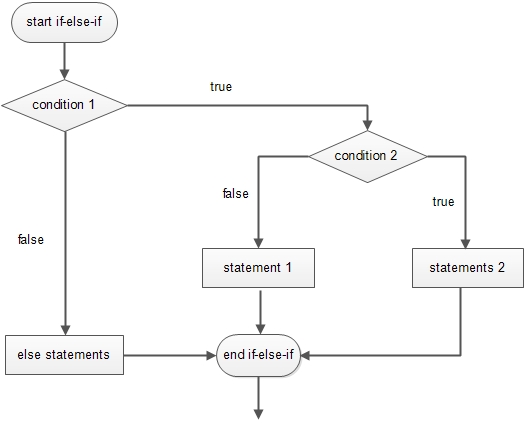
Example: Nested if statement in c++
//nested if else Statement in C++
#include <iostream>
using namespace std;
int main()
{
int number1, number2, number3;
cout<<"Enter first number"<<endl;
cin>>number1;
cout<<"Enter second number"<<endl;
cin>>number2;
cout<<"Enter third number"<<endl;
cin>>number3;
if(number1>number2)
{
if(number1>number3)
{
cout<<"Largest: "<< number1;
}
else
{
cout<<"Largest: "<<number3;
}
}
else
{
if(number2>number3)
{
cout<<"Largest: "<<number2;
}
else
{
cout<<"Largest: "<<number3;
}
}
return 0;
}
Output
Enter first number
5
Enter second number
8
Enter third number
3
Largest: 8