In this tutorial, you will learn about C++ Manipulators. They are used to styling output in various ways. This is the most common way to control the program output.
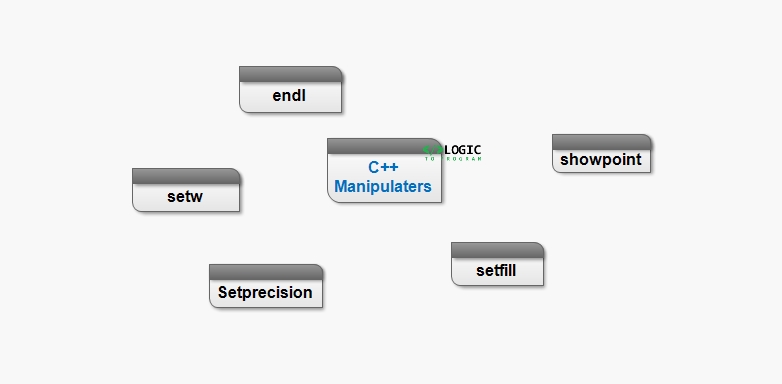
There are many manipulators used in the c++ language like, endl, setw, Setprecision, Setfill, Showpoint, and fixed.
Here we discuss them one by one.
endl manipulator
The manipulator endl is used to move the cursor to the beginning of the next line. It works like same as the working of \n escape sequence.
Example
Cout<<”Hello”<<endl<<”world”<<endl;
setw manipulator
This C++ manipulator is used to display output in specified columns. setw manipulator is the part of iomanip library.
Example
//setw manipulators in C++
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
cout<<"column1"<<setw(10)<<"column2"<<setw(12)<<"column3";
return 0;
}
Output
column1 column2 column3
setprecision manipulator
The setprecision manipulator is used to display a number of digits after the decimal point. Mostly it is used for the round of values. setprecision manipulator is the part of iomanip library.
Example
//Setprecision manipulators in C++
#include<iostream>
#include <iomanip>
using namespace std;
int main()
{
double a = 3.8978978;
cout << setprecision(5) << a <<endl;
cout << setprecision(7) << a <<endl;
cout << setprecision(3) << a <<endl;
}
Output
3.8979
3.897898
3.9
setfill manipulator
This manipulator is used to replace blank spaces with the specified characters. Setfill manipulator is effective with the use of setw. It is the part of iomanip library.
Example
//setfill manipulators in C++
#include<iostream>
#include <iomanip>
using namespace std;
int main()
{
double a = 3;
cout << setfill ('*') << setw (9);
cout << a << endl;
}
Output
********3
showpoint manipulator
The showpoint manipulator is used to write a decimal point for floating-point output even when it is not necessary.
Example
//Showpoint manipulators in C++
#include<iostream>
#include <iomanip>
using namespace std;
int main()
{
double a = 3;
cout << "With showpoint: " << showpoint << a <<endl;
cout<< "With noshowpoint: " << noshowpoint << a << endl;
}
Output
With showpoint: 3.00000
With noshowpoint: 3