Star pattern programs are very popular in programming languages to understand looping concepts. In this article, we will learn the C++ program to print star patterns using steric and loops.
Example 1: C++ program to print triangle or half pyramid
Triangle or a half pyramid is actually a right-angle triangle constructed by using the steric symbol. In this C++ program, take the maximum number of rows from the user and display a triangle or half pyramid on the console.
Code:
//C++ program to print triangle or half pyramid
#include <iostream>
using namespace std;
int main()
{
int i, j, rows;
cout << "Enter total number of rows: ";
cin >> rows;
for(i = 1; i <= rows; i++)
{
for(j = 1; j <= i; j++)
{
cout << "*";
}
cout << "\n";
}
return 0;
}
Output
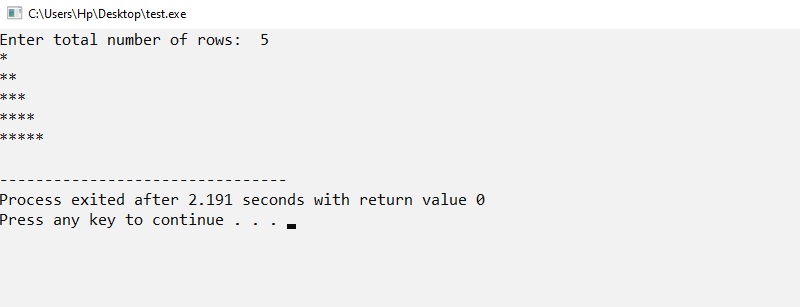
Example 2: C++ program to print inverted triangle or half pyramid
In this program, display the inverted triangle or half pyramid downward on the console scree. Check the program below.
//C++ program to print inverted triangle or half pyramid
#include <iostream>
using namespace std;
int main()
{
int i, j, rows;
cout << "Enter total number of rows: ";
cin >> rows;
for(i = rows; i >= 1; i--)
{
for(j = 1; j <= i; j++)
{
cout << "*";
}
cout << "\n";
}
return 0;
}
Output
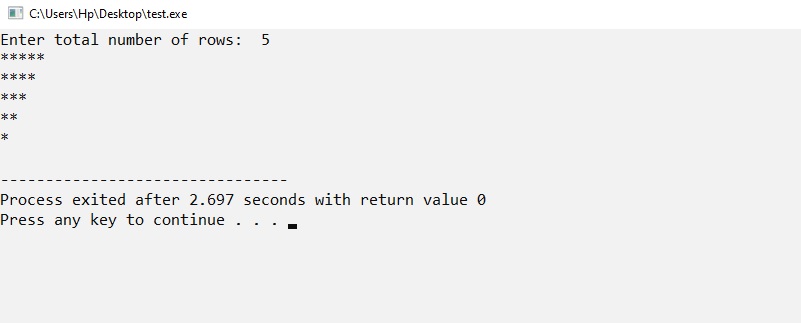
Example 3: C++ program to print full pyramid
The Bellow program helps you to display the full pyramid on the screen. Its design looks like historical Egyptian pyramids.
//C++ program to print full pyramid
#include<iostream>
using namespace std;
int main()
{
int i, j, rows, space;
cout << "Enter number of rows: ";
cin >> rows;
for(i = 1; i <= rows; i++)
{
for(space = i; space < rows; space++)
{
cout << " ";
}
for(j = 1; j <= (2 * i - 1); j++)
{
cout << "*";
}
cout << "\n";
}
}
Output

Example 4: C++ program to print inverted full pyramid
This program displays a full pyramid just inverted downward.
//C++ Program to print inverted full pyramid
#include<iostream>
using namespace std;
int main()
{
int i,j, rows, space;
cout << "Enter total number of rows: ";
cin >> rows;
for(i = rows; i >= 1; i--)
{
for(space = i; space < rows; space++)
cout << " ";
for(j = 1; j <= (2 * i - 1); j++)
cout << "*";
cout << "\n";
}
return 0;
}
Output

Example 5: C++ program to print diamond pattern
This program displays a rhombus-shaped diamond pattern.
//C++ program to print diamond pattern
#include<iostream>
using namespace std;
int main()
{
int i, j, rows, space;
cout << "Enter number of rows: ";
cin >> rows;
for(i = 0; i <= rows; i++)
{
for(space = rows; space > i; space--)
cout << " ";
for(j=0; j<i; j++)
cout << "* ";
cout << "\n";
}
for(i = 1; i < rows; i++)
{
for(space = 0; space < i; space++)
cout << " ";
for(j = rows; j > i; j--)
cout << "* ";
cout << "\n";
}
return 0;
}
Output
