In this article, you will learn a C++ program for simple calculator using switch statement. This program takes any of the operators (+, -. *, /) and two numbers from the user to perform addition, subtraction, multiplication, or division.
Let’s have a look if the user enters 5 and 8 as two numbers along with “+” as an operator. The logic looks like sum = 5 + 8 which is equal to 13.
Example: Simple calculator in C++
//C++ program for simple calculator
#include <iostream>
using namespace std;
int main()
{
char opr;
float number1, number2;
cout<<"Enter any operator from (+, -, *, /): ";
cin >> opr;
cout<<"Enter first number: ";
cin >> number1;
cout<<"Enter second number: ";
cin>> number2;
switch (opr) {
case '+':
cout <<"Addition of two numbers: "<< number1 + number2;
break;
case '-':
cout <<"Substraction of two numbers: "<< number1 - number2;
break;
case '*':
cout<<"Multiplication of two numbers: " << number1 * number2;
break;
case '/':
cout<<"Division of two numbers: " << number1 / number2;
break;
default:
cout << "Wrong Operator!!";
break;
}
return 0;
}
Output
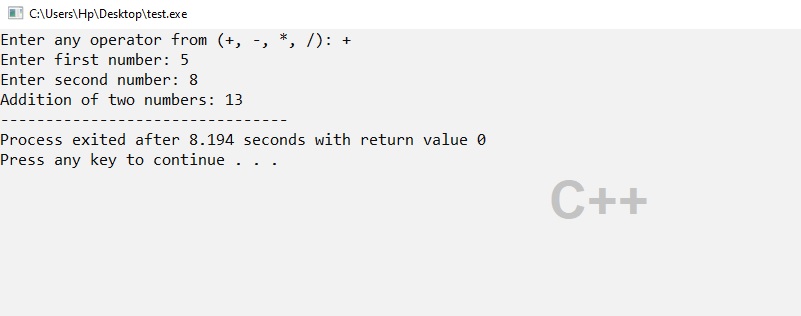