In this article, you will learn a C++ program to find the Transpose of a matrix using an array. Transpose can be calculated by interchanging matrix rows into columns and columns into rows.
Example: Find the transpose of a matrix in C++
This C++ program takes matrix size and its rows and columns elements from the user and displays its transpose on the console screen.
//C++ program for Transpose of matrix using arrays
#include <iostream>
using namespace std;
int main() {
int row, column, matrix[10][10], transposeMatrix[10][10];
cout << "Enter matrix total rows: ";
cin >> row;
cout << "Enter matrix total columns: ";
cin >> column;
cout << "\nEnter total number of elements: " << endl;
for (int i = 0; i < row; ++i) {
for (int j = 0; j < column; ++j) {
cout << "Enter element for a matrix position " << i + 1 << j + 1 << ": ";
cin >> matrix[i][j];
}
}
cout << "\nYour entered matrix will be : " << endl;
for (int i = 0; i < row; ++i) {
for (int j = 0; j < column; ++j) {
cout << " " << matrix[i][j];
if (j == column - 1)
cout << endl;
}
}
for (int i = 0; i < row; ++i)
for (int j = 0; j < column; ++j) {
transposeMatrix[j][i] = matrix[i][j];
}
cout << "\nThe Transpose of Matrix will be: " << endl;
for (int i = 0; i < column; ++i)
for (int j = 0; j < row; ++j) {
cout << " " << transposeMatrix[i][j];
if (j == row - 1)
cout<< endl;
}
return 0;
}
Output
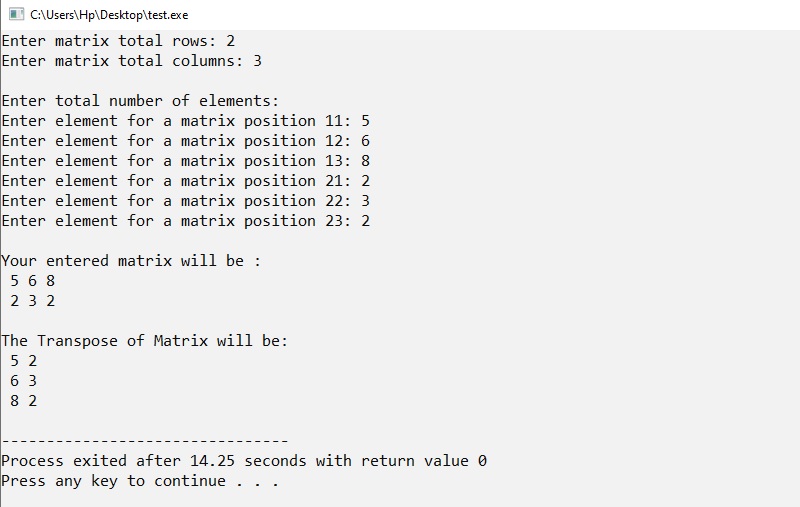