In this article, you will learn a C++ program for the subtraction of two matrices. The two matrices are added and subtracted if both have the same dimensions.
Example: Addition of two matrices in C++
In this program, we will use a multidimensional array to store the elements of matrices and perform subtraction. After subtraction of their corresponding elements display the result on the console screen.
//Subtraction of two matrices in C++
#include <iostream>
using namespace std;
int main()
{
int i, j, row, col, firstMatrix[50][50], secMatrix[50][50], result[50][50];
cout << "Enter number of rows from 0-50: ";
cin >> row;
cout << "Enter number of columns from 0-50: ";
cin >> col;
cout << endl << "Enter elements of first matrix: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << "Enter element for firstMatrix " << i + 1 << j + 1 << " : ";
cin >> firstMatrix[i][j];
}
cout << endl << "Enter elements of second matrix: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << "Enter element for secMatrix " << i + 1 << j + 1 << " : ";
cin >> secMatrix[i][j];
}
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
result[i][j] = firstMatrix[i][j] - secMatrix[i][j];
cout << endl << "Subtraction of two matrix will be: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << result[i][j] << " ";
if(j == col - 1)
cout << endl;
}
return 0;
}
Output
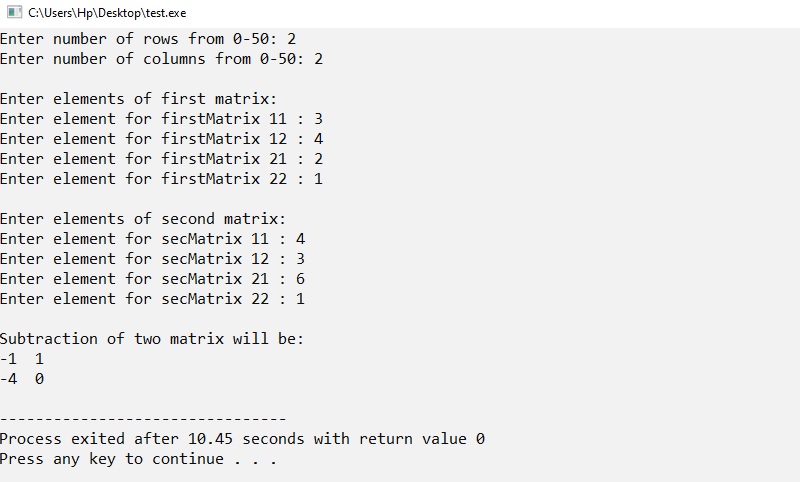