In this article, you will learn a C++ program for the addition of two matrices. The matrices are the arrangement of numbers into rows and columns. If we have a matrix having 2 rows and 3 columns. We can say that it’s a 2X3 matrix.
Two matrices are added if both have the same dimensions.
Example: Addition of two matrices in C++
In this program, we will use a multidimensional array to store the elements of matrices and perform addition. After adding their corresponding elements display the result on the console screen. Â
//Addition of two matrices in C++
#include <iostream>
using namespace std;
int main()
{
int i, j, row, col, firstMatrix[50][50], secMatrix[50][50], result[50][50];
cout << "Enter number of rows from 0-50: ";
cin >> row;
cout << "Enter number of columns from 0-50: ";
cin >> col;
cout << endl << "Enter elements of first matrix: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << "Enter element for firstMatrix " << i + 1 << j + 1 << " : ";
cin >> firstMatrix[i][j];
}
cout << endl << "Enter elements of second matrix: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << "Enter element for secMatrix " << i + 1 << j + 1 << " : ";
cin >> secMatrix[i][j];
}
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
result[i][j] = firstMatrix[i][j] + secMatrix[i][j];
cout << endl << "Sum of two matrix will be: " << endl;
for(i = 0; i < row; ++i)
for(j = 0; j < col; ++j)
{
cout << result[i][j] << " ";
if(j == col - 1)
cout << endl;
}
return 0;
}
Output
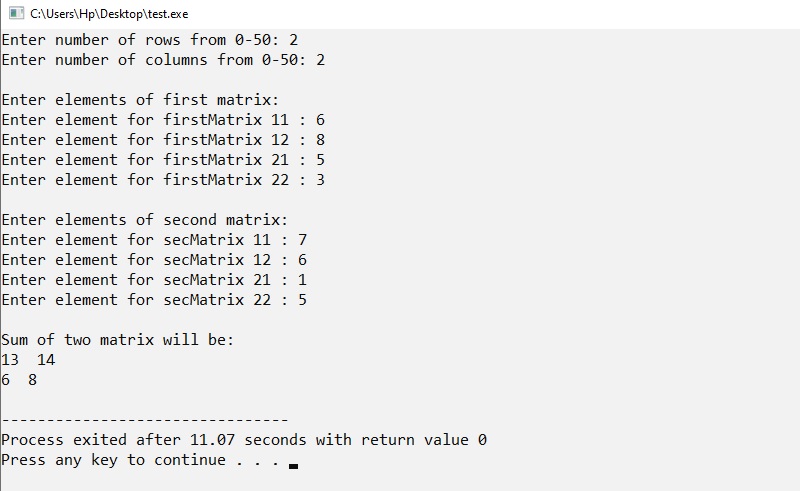