In this article, you will learn a C++ program to check prime numbers. The concept of prime numbers is very clear. All the positive integers that are divisible by 1 and themselves are called prime numbers.
If we have a number 17. It’s a prime number because it is divisible by 1 and itself. While on the other hand 25 is not a prime number as it is divisible by 1, 5, and 25.
The list of the first 10 prime numbers are:
2, 3, 5, 7, 11, 13, 17, 19, 23, 29
Here we discuss two different examples to check prime numbers in C++.
- Check prime numbers in C++
- Display prime number between two intervals
Example 1: Check prime numbers in C++
In this example, the program takes any number from the user and finds whether the entered number is prime or not.
//C++ program to check prime numbers
#include<iostream>
using namespace std;
int main()
{
int number, i, check=0;
cout<<"Enter Any Number: ";
cin>>number;
for(i=2; i<number; i++)
{
if(number%i==0)
{
check++;
break;
}
}
if(check==0)
cout<<number<<" is a Prime Number";
else
cout<<number<<" is not a Prime Number";
cout<<endl;
return 0;
}
Output
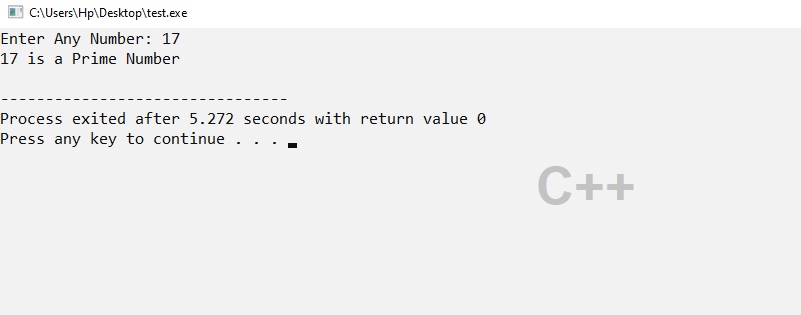
Example 2: Display prime numbers between two intervals
In this example, the program takes two intervals from the user, and the program displays all the prime numbers between these intervals. Check the code below.
//C++ program to check prime numbers between two intervels
#include<iostream>
using namespace std;
int main()
{
int intervel1, intervel2, num, i, j;
cout<<"Enter First Intervel: ";
cin>>intervel1;
cout<<"Enter Second Intervel: ";
cin>>intervel2;
cout<<"Prime Numbers between "<<intervel1<<" and "<< intervel2<<" are";
for (i = intervel1 + 1; i < intervel2 ; ++i){
num = 0;
for (j = 2; j <= i/2; ++j){
if (i % j == 0){
num = 1;
break;
}
}
if (num == 0){
cout<<"\n"<<i;
}
}
return 0;
}
Output
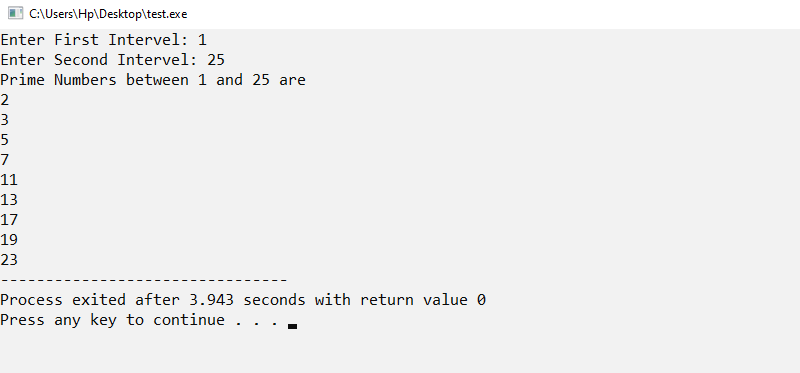