In this article, you will learn a C++ program to find the factorial of any number. This program takes a number as input from the user and displays its factorial on the console screen.
Factorial can be calculated by multiplying the factorial value with its previous positive integers. You can check the formula below.
Formula
n! = n*(n-1)*(n-2)….*1
Example 1
In this example, We shall compute the factorial of 5. The number is always followed by an exclamation mark, such as “5!”
5! = 5*4*3*2*1 = 120
Example 2: C++ program to find factorial using for loop
Here the factorial can be calculated by using for loop. Check the program below
//C++ program to find factorial
#include <iostream>
using namespace std;
int main() {
int n, factorial = 1.0;
cout << "Enter any positive integer: ";
cin >> n;
for(int i = 1; i <= n; ++i) {
factorial *= i;
}
cout << "Factorial of " << n << " = " << factorial;
return 0;
}
Output

Example 3: Find factorial by using recursive function
Now let’s check the factorial by using a recursive function. You can check the program below.
//C++ program to find factorial using recursive function
#include <iostream>
using namespace std;
int fact(int n);
int main() {
int n;
cout << "Enter any positive integer: ";
cin >> n;
cout << "Factorial of " << n << " = " << fact(n);
return 0;
}
int fact(int n) {
if ((n==0)||(n==1))
return 1;
else
return n*fact(n-1);
}
Output
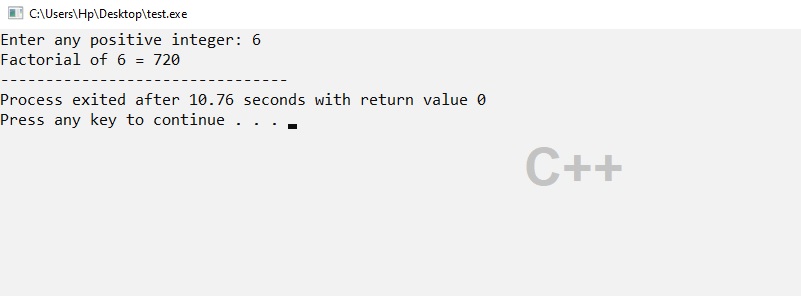