In this article, you will learn a C++ program to check the alphabet. This program takes any character from the user and the program tells whether the entered character is an alphabet or not.
Here we discuss two different approaches to checking the alphabet in C++.
- Using the if-else statement
- Using ASCII values
Let’s check the above approaches one by one.
Example 1: Check Alphabet in C++ Using if-else statement
In this example, the “if else” statement is used to evaluate the condition. If the user entered a character greater than or equal to “A” or less than or equal to “Z” display your entered character is an alphabet otherwise display it’s not an alphabet. Similarly checks the condition for lower case letters. You can check the code below
Code
//C++ program to check the alphabet
#include<iostream>
using namespace std;
int main()
{
char chr;
cout<<"Enter any Character: ";
cin>>chr;
if((chr>='a' && chr<='z') || (chr>='A' && chr<='Z'))
{
cout<<"\n"<<chr<<" is an Alphabet \n";
}
else
{
cout<<"\n"<<chr<<" is not an Alphabet";
}
return 0;
}
Output
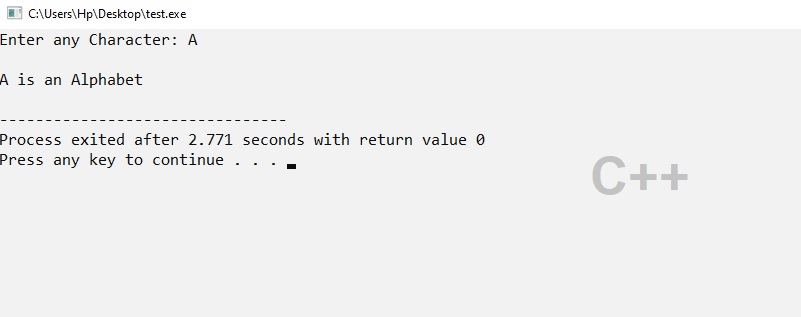
Example 2: Check Alphabet in C++ using ASCII numbers
Here is another C++ program to check the alphabet. ASCII numbers are used to check the condition.
The ASCCI values for uppercase are in the range of 65 to 90 and for the lower case range from 97 to 122. All the values in between these ranges are alphabet otherwise not an alphabet.
Code
#include<iostream>
using namespace std;
int main()
{
char chr;
cout<<"Enter any Character: ";
cin>>chr;
if((chr>=65 && chr<=90) || (chr>=97 && chr<=122))
{
cout<<"\n"<<chr<<" is an Alphabet \n";
}
else
{
cout<<"\n"<<chr<<" is not an Alphabet";
}
return 0;
}
Output
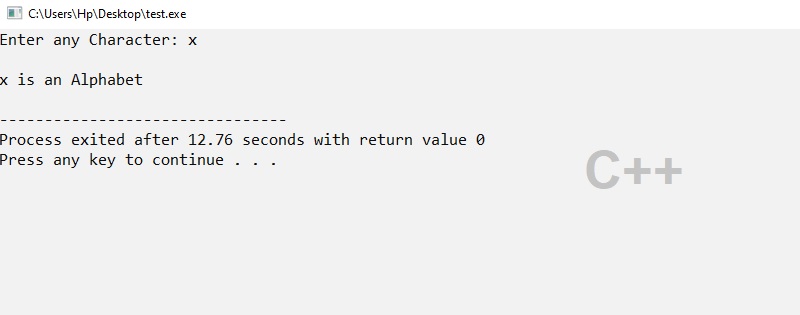