You declare a variable after that operators are used to perform logical or mathematical operations on that variable’s values. For example, int age = 27; where the “=” symbol is an assignment operator that assigns 27 to the age variable.
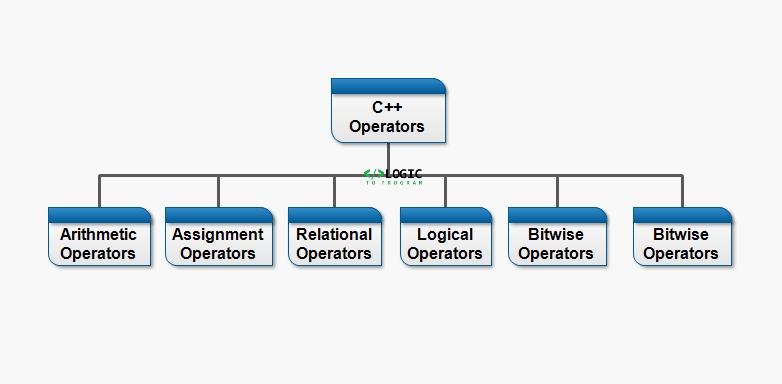
C++ programming language support many operators that are divided into different groups.
- Arithmetic operators
- Assignment operators
- Relational operators
- Logical operators
Let’s discuss them one by one.
1. Arithmetic operators
It is a well-known group of operators to perform a mathematical operation on variables. Here is the list of arithmetic operators along with their operational effects.
Operator | Name | Description | Example |
+ | Addition | Used to add two values | Num1+num22 |
– | Subtraction | Subtract one value from the other | num1-num2 |
* | Multiplication | Multiplying two values | Num21*num2 |
/ | Division | Divide two values | num1/num2 |
% | Modules | Used to get division reminder | Num1%num2 |
Example Arithmetic operators
//Arathmatic operators
#include<iostream>
using namespace std;
int main()
{
int num1=8, num2=2;
cout<<"num1 + num2 = "<<num1+num2<<endl;
cout<<"num1 - num2 = "<<num1-num2<<endl;
cout<<"num1 * num2 = "<<num1*num2<<endl;
cout<<"num1 / num2 = "<<num1/num2<<endl;
cout<<"num1 % num2 = "<<num1%num2<<endl;
}
Output
num1 + num2 = 10
num1 - num2 = 6
num1 * num2 = 16
num1 / num2 = 4
num1 % num2 = 0
2. Assignment Operators
As its name indicated that they are used to assign value to the variables.
Let’s have look at different assignment operators with examples.
Operator | Name | Equals to |
= | num1= num2 | Num1 = num2 |
+= | num1+=num2 | num1 = num1+num2 |
-= | num1-=num2 | num1 = num1-num2 |
*= | num1*=num2 | num1 = num1*num2 |
/= | num1/=num2 | num1 = num1/num2 |
%= | num1%=num2 | num1 = num1%num2 |
Example Assignment Operators
//Assignment operator
#include<iostream>
using namespace std;
int main()
{
int num1=8, num2=2;
num1+=num2;
cout<<"num1 += num2 = "<<num1<<endl;
}
Output
num1 += num2 = 10
3. Relational Operators
Relational c++ operators are also known as comparison operators used to compare two values. They check the condition of both values and give “true as 1” or “false as 0” results depending on the relational operator used.
Here is the list of relational operators with examples
Operator | Name | Example |
== | Equals to | 4==5 give result false |
!= | Not equal to | 4!=5 gives the result true |
< | Less then | 4<5 give result true |
> | Greater then | 4>5 gives the result false |
<= | Less than equal to | 4<=5 give result false |
>= | Greater than equal to | 4>=5 give result false |
Example Relational Operators
//Relational operator
#include<iostream>
using namespace std;
int main()
{
int num1=8, num2=2;
int result;
result = (num1 == num2); // false
cout << "8 == 2 is " << result << endl;
result = (num1 != num2); // true
cout << "8 != 2 is " << result << endl;
result = (num1 > num2); // false
cout << "8 > 2 is " << result << endl;
result = (num1 < num2); // true
cout << "8 < 2 is " << result << endl;
result = num1 >= num2; // false
cout << "8 >= 2 is " << result << endl;
result = (num1 <= num2); // true
cout << "8 <= 2 is " << result << endl;
}
Output
8 == 2 is 0
8 != 2 is 1
8 > 2 is 1
8 < 2 is 0
8 >= 2 is 1
8 <= 2 is 0
4. Logical operators
Logical operators in c++ are used to check whether the condition is true or false. They are commonly used with conditional statements.
Operator | Name | Example | Description |
&& | And operator | Num1 && num2 | True when both num1 and num2 are true otherwise give a false result |
|| | Or operator | Num1 || num2 | True when num1 or num2 is true else give a false result |
! | Not operator | !num | True when num is false |