C++ Data Types tell about the size and the kind of data that is being stored in the variable. Each data type requires a different amount of memory based on its type.
For example, int age = 27; where int is the data type that tells integer value stored in the variable “age” and takes 2 or 4 bytes in the memory location.
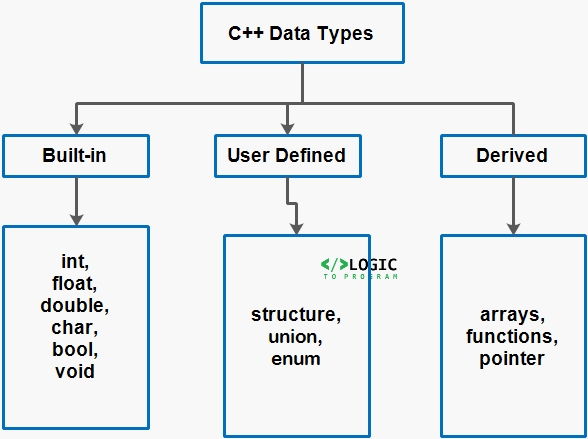
C++ Built-in Data Types
Here is a list of Built-in data types that are most frequently used in C++ programming.
Data Type | Size | Description |
void | 0 bytes | It means empty or none |
bool | 1 bit | Used to store true or false value (T/F) |
char | 1 bit | It stores only a single character(alphabet or a number) |
int | 2 or 4 bytes | Store non-decimal whole numbers |
float | 4 bytes | It is used to store decimal numbers up to 7 decimal places |
double | 8 bytes | Used to store decimal numbers up to 15 decimal places |
Let’s briefly discuss C++ built-in data types one by one
Void in C++
The void keyword is used for no value or nothing in the variable. It requires 0 bytes in the memory space. It is mostly used in functions that do not return any value.
Void sum()
Bool in C++
Bool is a keyword used to store Boolean values (T/F) true or false. It takes only 1 byte on the memory space. Mostly used in conditional statements.
bool graduated = true;
char in C++
char keyword is used to store a single character, a number, or ASSCI values. It takes only 1 byte of memory. char values are always enclosed in a single quote (”).
char Value = 'A'
Int in C++
Int is a keyword used to store an integer value in a variable. It takes 2 or 4 bytes of memory depending upon the system architecture type. It can store values ranging from “-2147483648 to 2147483647”.
Int marks = 90;
Float in C++
Float named as floating-point used to store fractional or decimal values. It usually takes 4 bytes on the computer’s memory.
float height =5.9;
Double in C++
Double is also used for storing fractional or decimal values. It stores larger values because it takes more memory (8 bytes) than the floating point.
Double speed = 95.9874